Usage and Manipulation of Variables
Variables are tightly integrated into the whole Better EHR Studio structure and you can use them in different areas.
The table below shows where you can use each type of variable.
usage \ variable type | static variable | externally/internally defined | AQL variable |
---|---|---|---|
dependencies when clause | ✅ | ✅ | ⬜️ |
dependencies then clause | ⬜️ | ✅ | ⬜️ |
expression | ✅ | ✅ | ✅ |
rich text | ✅ | ✅ | ⬜️ |
html element | ✅ | ✅ | ⬜️ |
aql building | ⬜️ | ✅ | ⬜️ |
column/stacked list valueset | ⬜️ | ⬜️ | ✅ |
dropdown inputs | ⬜️ | ⬜️ | ✅ |
javascript API | ✅ | ✅ | ✅ |
Some practical examples:
When / Then Clauses
When a clause is a condition clause, something else will happen when something is in a given state.
A quintessential example would be:
When isFormPrefilled
equals true
,
then date stored
is shown.
With when / then clauses, you add interactivity to the form. You can see how the variables change and then change something else in the form according to a specific variable value.
Expressions
Expressions help calculate and transform data, e.g., format date, BMI formula, and concatenating text.
Using Variables in Dependency When / Then Clauses
Static Variable in a When Clause
The above example shows how to display a warning message with rich text and show it only when a form is in read-only mode.
The 'when' clause in the example is:
When isReadOnly
equals true,
then warning
show,
otherwise warning
hide.
The result of the interaction is a warning that is displayed when you put a form into read-only mode.
Internally Defined Variable in a Then Clause
When you want to change a value of a variable based on user input, you can use the 'then' clause. For example, you would use it to calculate a patient score based on the patient data.
In the example below, we calculated the score based on the patients BMI, unplanned weight loss and presence of an acute disease:
NOTE: Static and AQL variables cannot be changed. The values of static and AQL variables are omitted, because the clause would change otherwise. Even though the definition of an externally defined variable is that it comes from outside the Form renderer, you can still change its value inside the form.
Internally Defined Variable in a When Clause
Based on the calculated score, you can now show the appropriate risk-rich text.
For example:
- if the score is 0, then you show Low risk rich text,
- if the score is 1, then you show Medium risk rich text, and
- if the score is 2, then you show High risk rich text.
Now, based on the patient data, the form alerts the doctor of the health risk.
Using Variables in Expressions
Expressions help you format and manipulate data. You can find expressions in different locations of the Form Builder.
NOTE: Writing expressions is easier with Expression Assistant. Visit the Expression Assistant documentation to learn more.
Static Variable Expression
In the above example, you use the currentDateTime variable in a 'then' clause, and then use the expression to format the date.
Internal Variable Expressions
In the same way, you can add expressions to internally defined variables.
In the above example, we calculated the internally defined variable HEART_1 by adding the History, EKG, and Age values.
AQL Variable Expressions
AQL variables get its value from an AQL query result.
The picture shows weight_aql variable example that reads the body weight from a composition.
You can also manipulate the value of an AQL variable with an expression.
The example shows how you can use 'when' and 'then' clauses to calculate BMI from a patient's weight when weight is obtained through an AQL.
Using Variables in Generics
Rich Text and HTML Elements
Both Rich text and HTML elements use a Mustache templating engine, and you can use the defined variables inside this generic.
The example shows the currentDate
static variable and an internally defined variable score
inserted into Rich text.
Please, visit the Rich text documentation and HTML element documentation to learn more.
Column / Stacked List Valueset
Column / stacked list lets you display an AQL result in a list. For more information, please visit the Column list documentation.
In the example above, there is a column list showing the results from the weight_patient_aql variable. Weight_patient_aql variable executes an AQL query that reads weight from compositions.
Columns displaying results can use expressions. You can add expressions to the label input.
For example, you can concatenate the bodyweight column to show both the magnitude and unit.
You can also format the date of the weight measurement.
Dropdown Inputs
Dropdown inputs have a label and a value. Both can use an expression.
The example shows a dropdown input that displays data from an AQL variable. The label has a FORMAT_DATE
expression, and the value displays the weight magnitude.
JavaScript API
The following example shows how to execute AQL and set a field value.
When the Manual input checkbox is ticked, the form is emptied and inputs appear. Otherwise, the form is filled with variable values and inputs are disabled.
An example of a code:
api.addListener('generic-boolean-xxxxx', 'CHANGE', function(model) {
let date = api.getVariable('currentDate').value;
let patient = api.getVariable('ehrId').value;
let weight_aql = api.getVariable('weight_aql').value.code;
var value = api.getFieldValue('generic-boolean-xxxxx');
if (value === true) {
api.clearFormElement('patient_id');
api.enableFormElement('patient_id');
api.clearFormElement('patient_weight');
api.enableFormElement('patient_weight');
api.clearFormElement('date');
api.enableFormElement('date');
} else {
api.setFieldValue('patient_id', patient);
api.disableFormElement('patient_id');
api.setFieldValue('date', date);
api.disableFormElement('date');
api.executeAql(weight_aql).then( data => api.setFieldValue('patient_weight', data.resultSet[0].weight, 'magnitude'));
api.disableFormElement('patient_weight');
}
})
Using openEhrUrl External Variable in API Connectors
Introduction
This is a step-by-step guide for creating an API connector to retrieve data from an openEHR server using a specified AQL view. The API connector will use the openEhrUrl variable to dynamically construct the URL needed for API calls.
Prerequisites
- Ensure you have an AQL view created in the AQL Builder. Refer to the AQL Builder documentation if you need assistance.
- You need the base URL of your openEHR server. For this example, we will use https://my-openEHR-server.com/rest/v1.
Before we Start
- The AQL view we will use is called
systolic-diastolic
. - The base URL of the openEHR server for this example is https://my-openEHR-server.com/rest/v1.
- The complete URL for the AQL view is https://my-openEHR-server.com/rest/v1/view/systolic-diastolic.
Step-by-step Guide
Step 1: Set the Test Value of the openEhrUrl Variable
First, you need to set the test value for the openEhrUrl variable to the URL of the openEHR server. This variable will be used to construct the full URL for API calls.
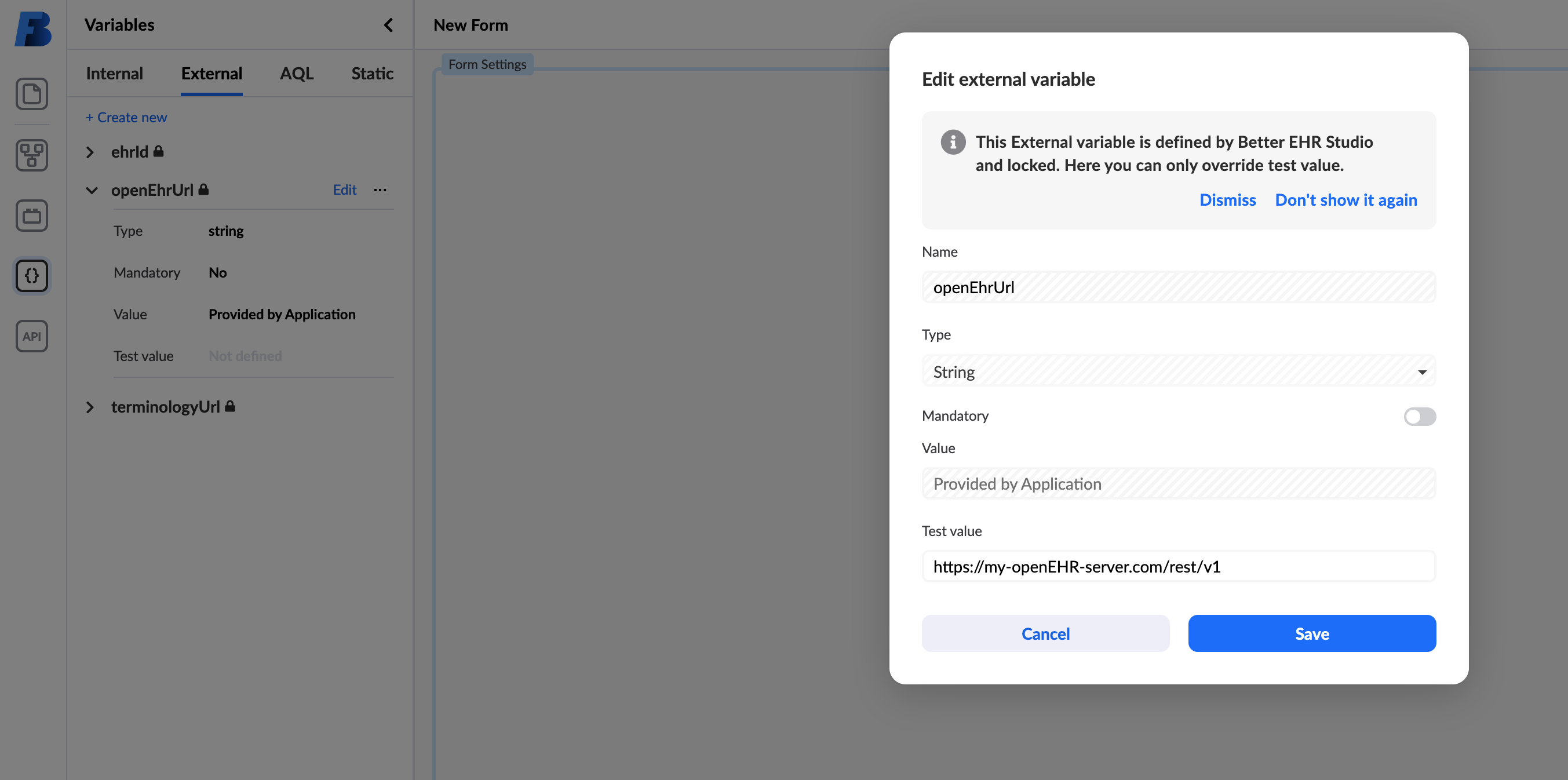
Step 2: Create an API Connector
- Create an API Connector: Add a new API connector to your form.
- Set the Base URL: Configure the base URL of the API connector using the openEhrUrl variable and the text
/view/
.
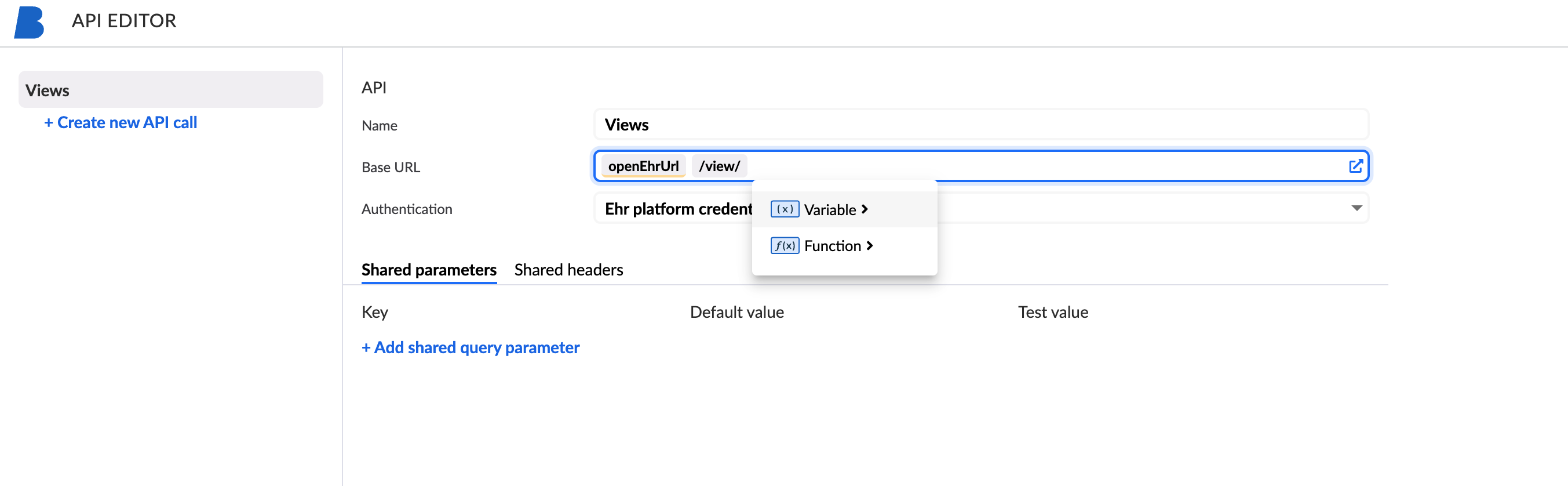
Step 3: Add an API Call
- Add API Call: Within the API connector, create a new API call.
- Set the Endpoint: Use
systolic-diastolic
as the specific endpoint. This will complete the URL.
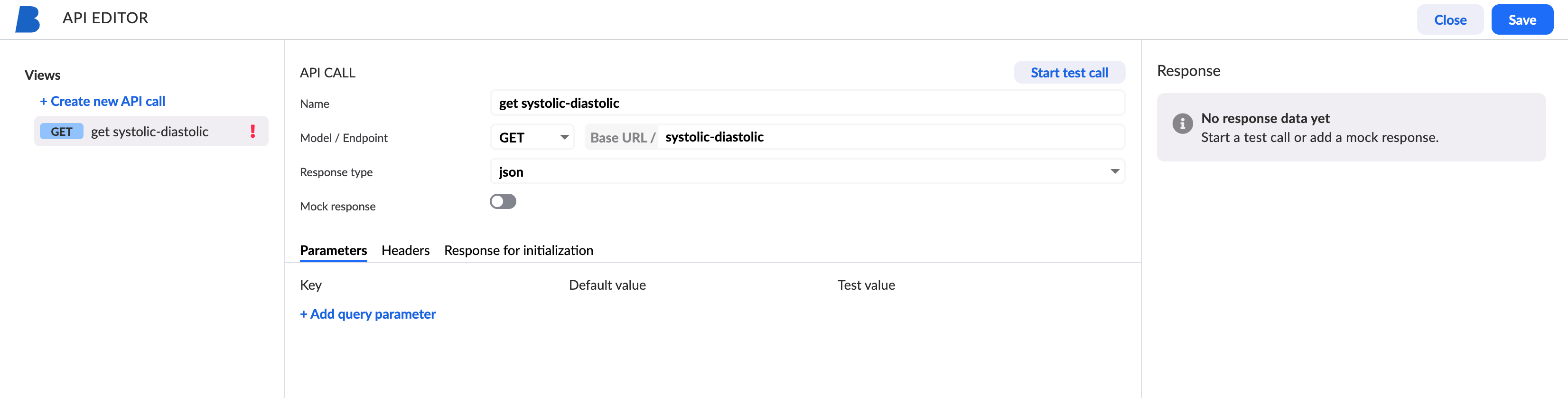
Step 4: Start Test Call
- Start Test Call: Start the test call to verify that the API connector can successfully retrieve data from the AQL view.
- Check Results: If the call is successful, you should see the data from the
systolic-diastolic
displayed in the right panel of the API connector.
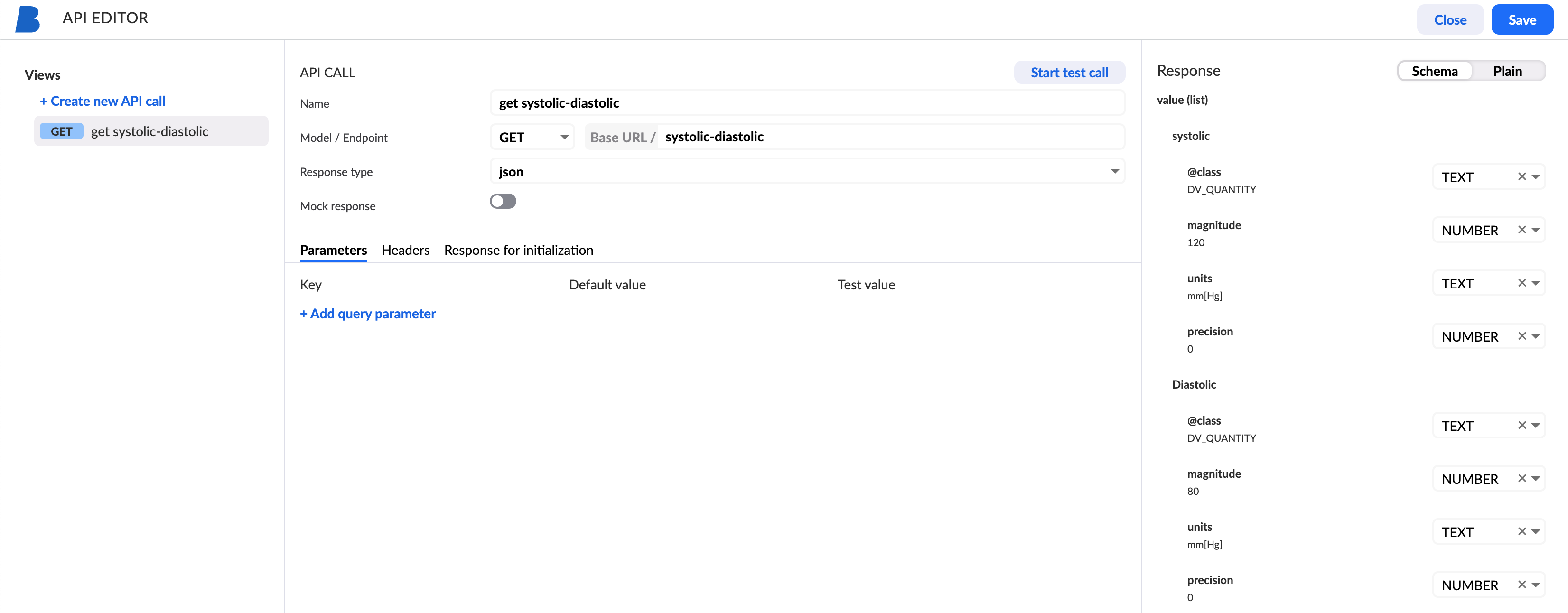
Troubleshooting
If you encounter issues during the test call, consider the following:
- Verify the definition of the AQL view.
- Ensure the test value for
openEhrUrl
variable is set to the correct openEHR server URL. - Double-check the configuration of the API connector.
- Check if your view requires any additional variables (for example ehrId) and make sure to pass that into the call and provide a test value for it
Once your test call is successful, your API connector is correctly configured and ready for use.